Welcome to an exciting journey through advanced coding techniques that will elevate your programming skills to a professional level.
From mastering algorithms to effective debugging strategies, each technique is designed to enhance your coding prowess and efficiency.
Whether you’re a seasoned coder or just stepping into the advanced realm, these tips will provide you with the knowledge to tackle complex challenges and impress your peers.
1. Mastering Data Structures
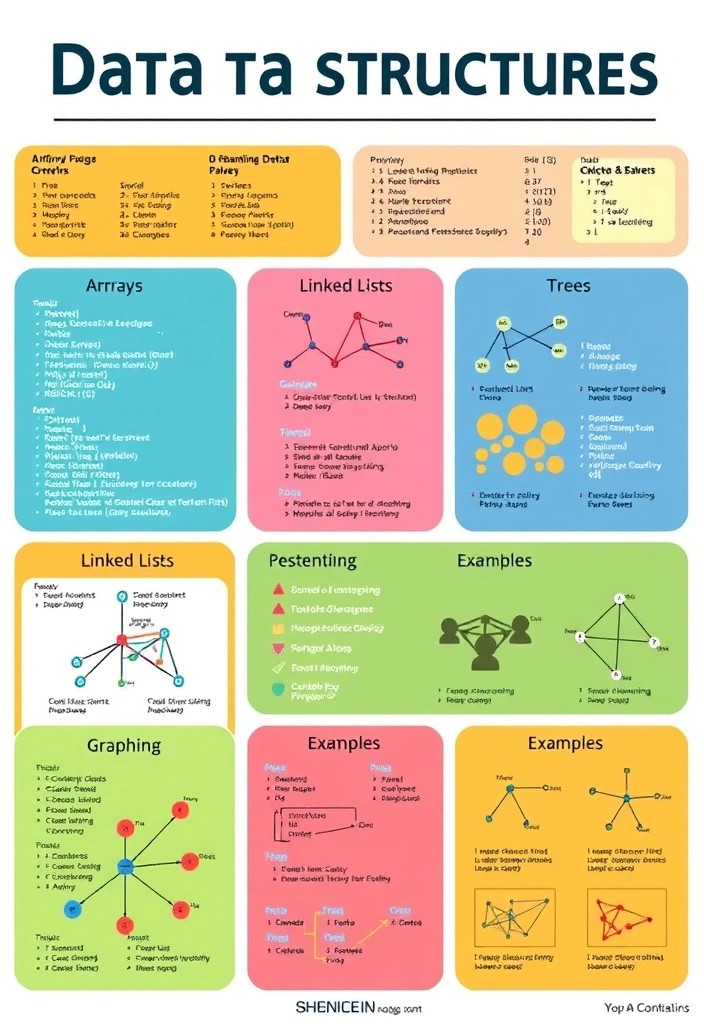
Understanding data structures is fundamental to becoming an advanced coder.
From arrays to linked lists, and trees to graphs, each data structure serves a unique purpose and can optimize your algorithms.
Mastering these concepts allows you to write more efficient code, manage memory effectively, and solve complex problems with ease.
Invest time in understanding the intricacies of each structure and practice implementing them in different programming languages.
Product Recommendations:
• Data Structures and Algorithms Made Easy by Narasimha Karumanchi
• Cracking the Coding Interview by Gayle Laakmann McDowell
• Introduction to Algorithms by Thomas H. Cormen
2. Advanced Algorithm Techniques
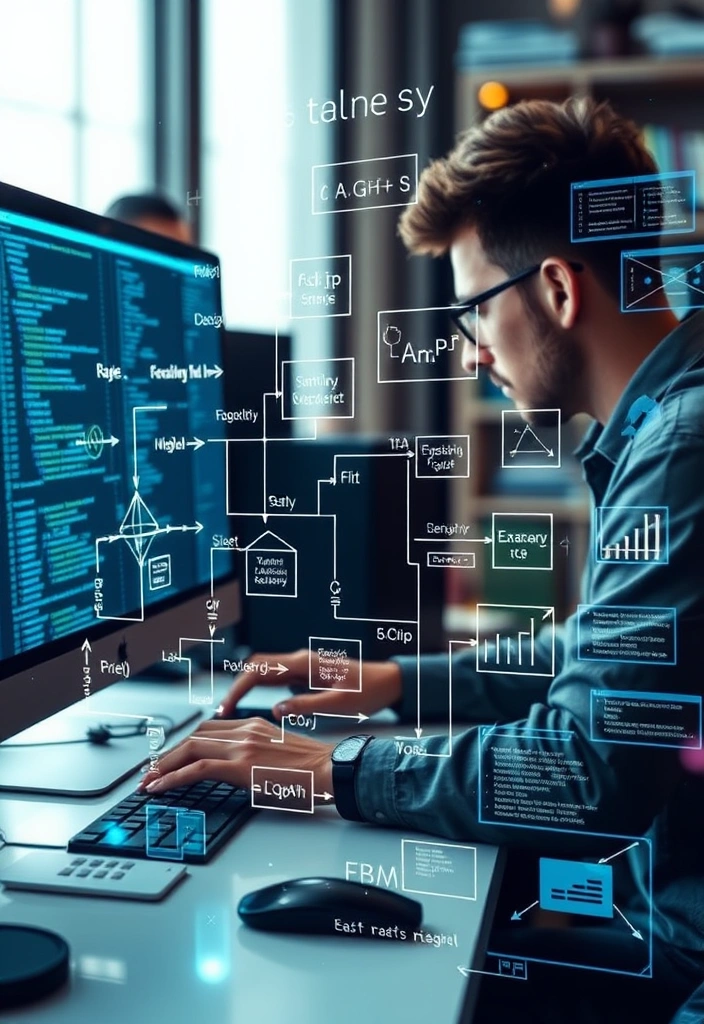
Algorithms are the backbone of programming, and mastering advanced techniques can set you apart.
Explore dynamic programming, greedy algorithms, and backtracking methods to solve complex problems efficiently.
Understanding the underlying principles of these techniques can help you optimize performance and reduce computation time in your applications.
Practice implementing these algorithms in real-world scenarios for a stronger grasp of their applications.
Product Recommendations:
• Introduction to Algorithms by Thomas H. Cormen
• Grokking Algorithms by Aditya Bhargava
• Algorithm Design Manual by Steven S. Skiena
3. Clean Code Practices
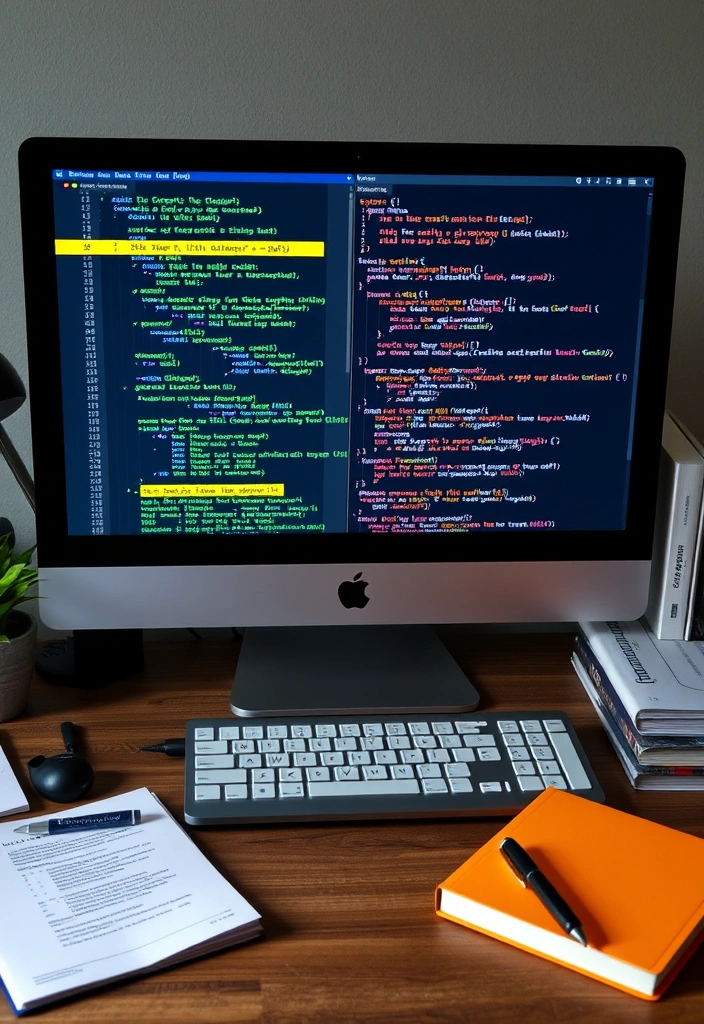
Writing clean code is essential for maintainability and collaboration.
Adopt practices such as meaningful naming conventions, consistent indentation, and thorough commenting.
Clean code not only improves readability but also helps you and others understand your logic in the future.
Invest in tools that can assist with code formatting and validation to keep your codebase tidy.
Product Recommendations:
• Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin
• Refactoring: Improving the Design of Existing Code by Martin Fowler
• The Clean Coder by Robert C. Martin
4. Version Control Mastery
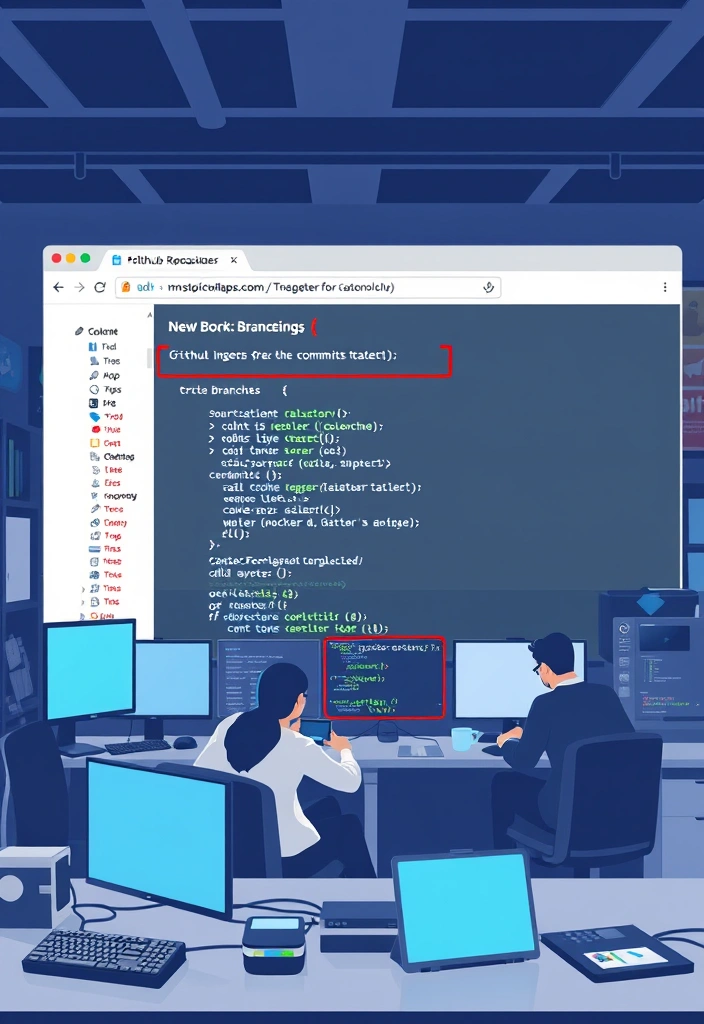
Version control is a vital skill for any professional developer.
Master Git and understand branching strategies, merging, and rebasing to manage your code effectively.
Learning version control not only helps you track changes but also allows for seamless collaboration with other developers.
Utilizing platforms like GitHub can enhance your workflow and provide a portfolio of your coding projects.
Product Recommendations:
• Pro Git by Scott Chacon and Ben Straub
• Git Pocket Guide by Richard E. Silverman
5. Testing and Debugging Techniques
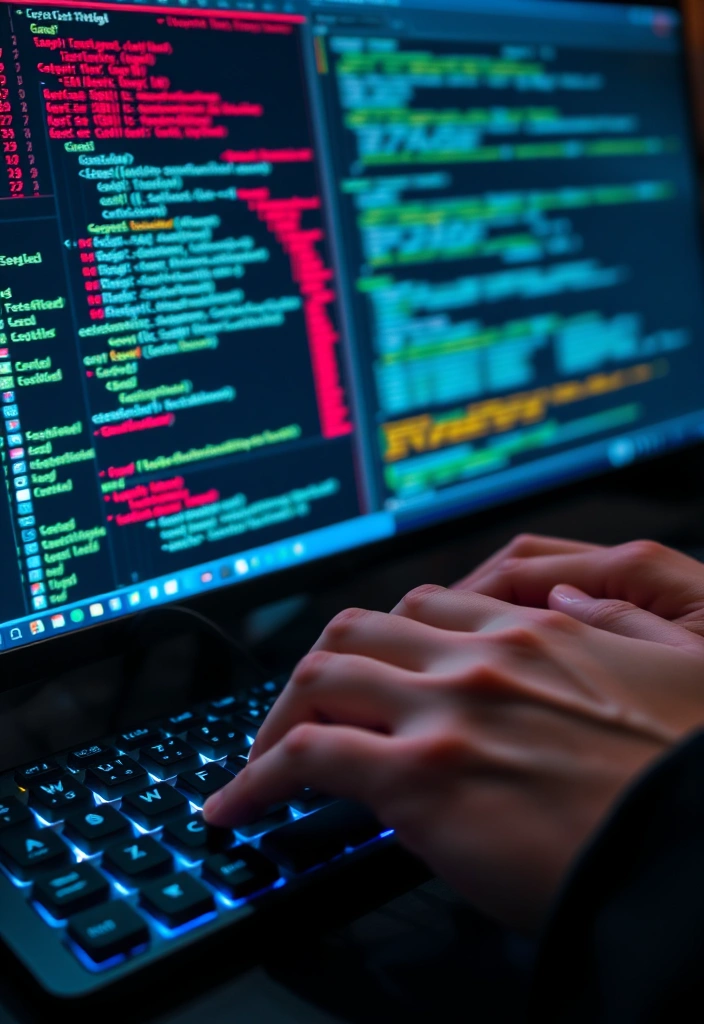
Advanced coding isn’t just about writing code; it’s also about ensuring it works flawlessly.
Learn unit testing, integration testing, and debugging techniques to catch errors early.
Implementing a robust testing strategy can save you time and effort by preventing bugs from reaching production.
Tools like Jest, Mocha, and Selenium can aid in automating your testing process.
Product Recommendations:
• Test-Driven Development with Python by Harry Percival
• The Art of Unit Testing by Roy Osherove
• JavaScript Testing Best Practices by Daniel Rosenwasser
6. Understanding Design Patterns
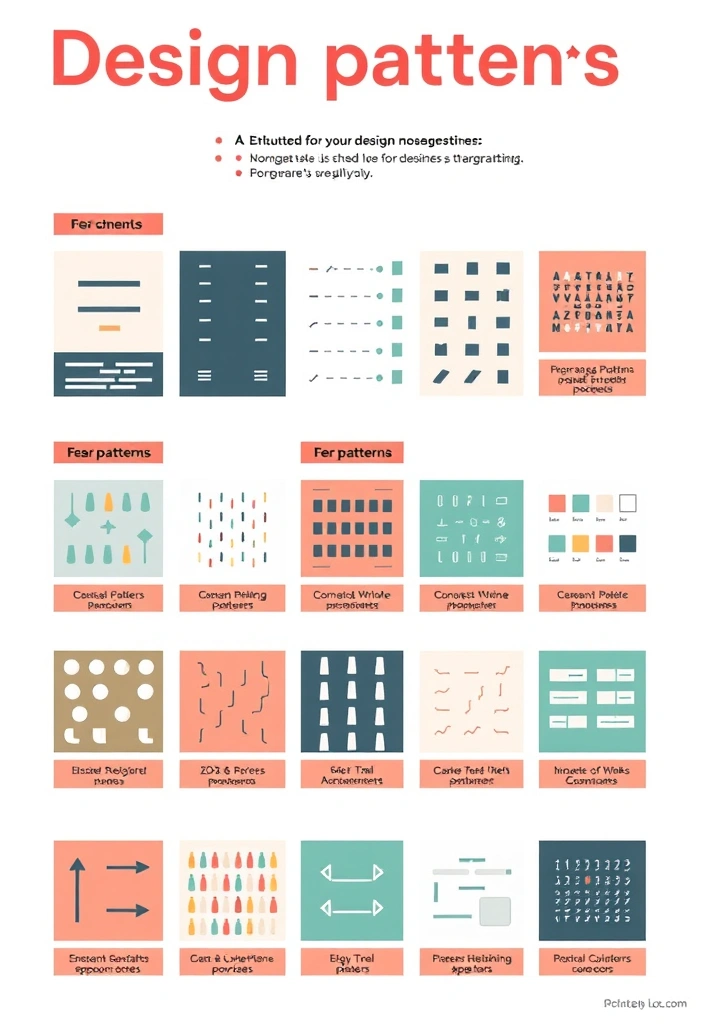
Design patterns are proven solutions to common problems in software design.
Familiarize yourself with patterns like Singleton, Observer, and Factory to enhance your software architecture.
Knowing when and how to apply these patterns can lead to more scalable, maintainable, and flexible code.
Study each pattern’s use cases and practice implementing them in your projects.
Product Recommendations:
• Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma
• Head First Design Patterns by Eric Freeman
• Design Patterns in Modern C++ by Dmitri Nesteruk
7. Asynchronous Programming
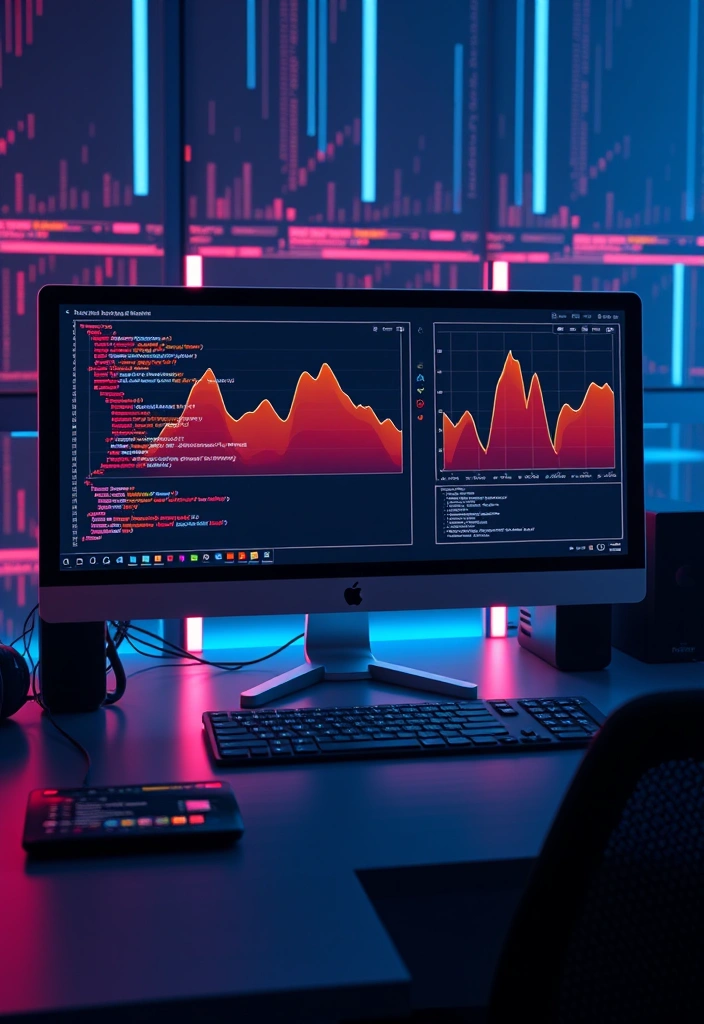
Asynchronous programming allows your code to run multiple tasks simultaneously, optimizing performance.
Learn about Promises, async/await syntax, and callbacks to manage asynchronous operations effectively.
Understanding these concepts can significantly improve the user experience and responsiveness of your applications.
Experiment with real-time data fetching and handling to see the benefits in action.
Product Recommendations:
• You Don’t Know JS Yet by Kyle Simpson
• Eloquent JavaScript by Marijn Haverbeke
• Async Programming in C# by Alex Davies
8. Code Review Best Practices
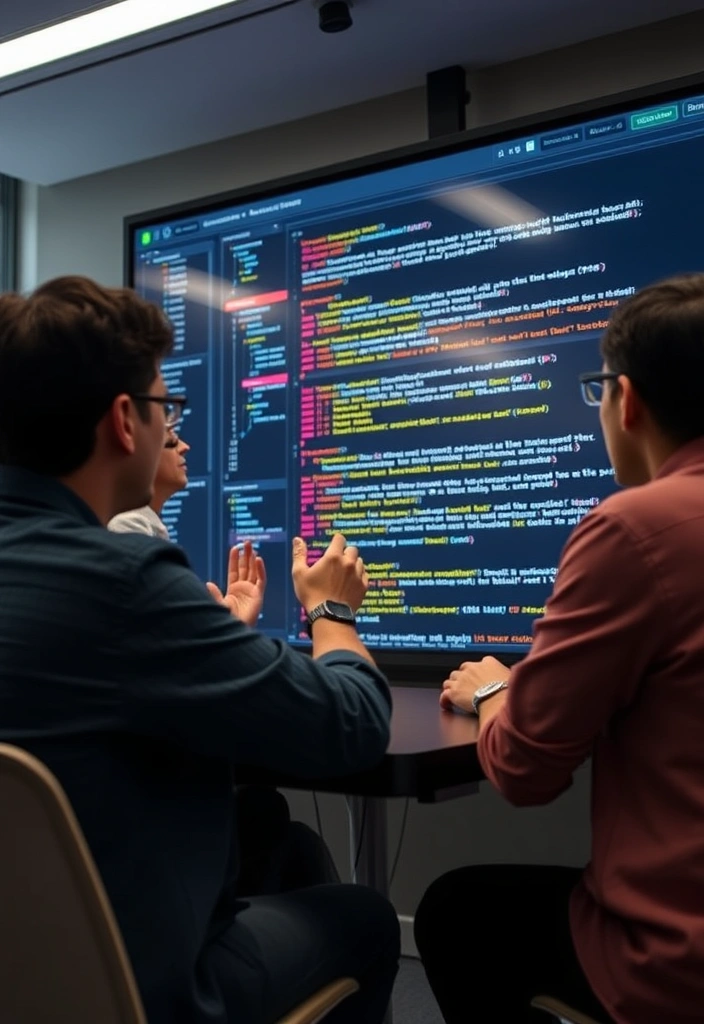
Participating in code reviews is a valuable way to learn from peers and improve your coding skills.
Understand how to give and receive constructive feedback, focusing on the logic and efficiency of the code.
Code reviews foster collaboration and can lead to the discovery of better solutions.
Make it a habit to participate in regular reviews and discussions about code quality.
Product Recommendations:
• Code Complete by Steve McConnell
• Working Effectively with Legacy Code by Michael C. Feathers
• The Phoenix Project by Gene Kim
9. Continuous Integration and Deployment
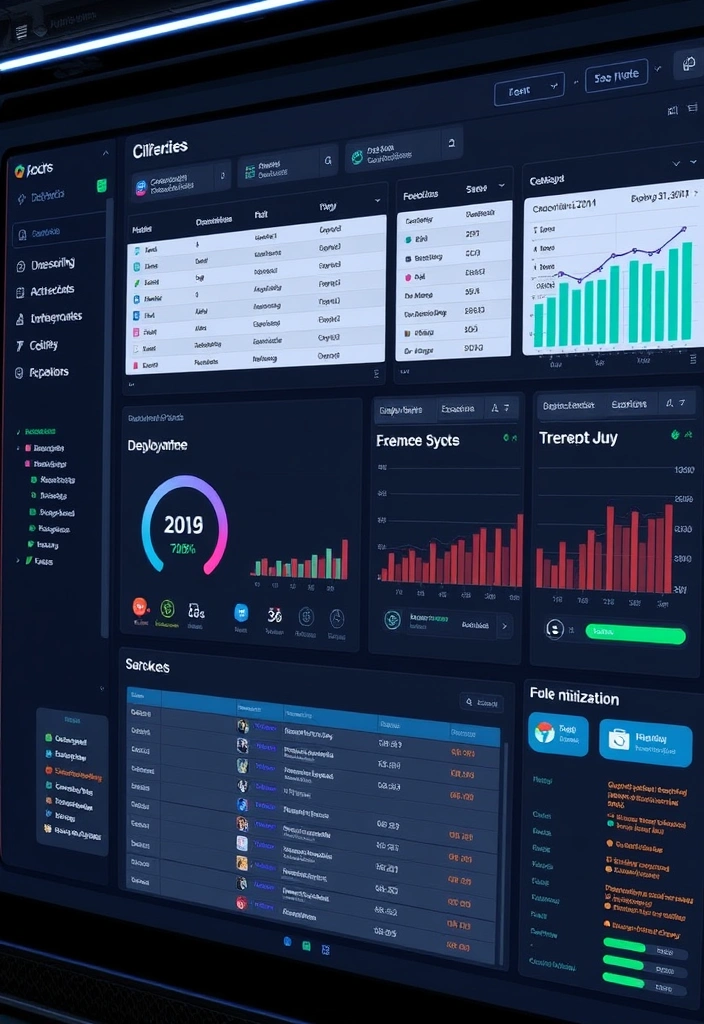
Continuous integration (CI) and continuous deployment (CD) are crucial for modern software development.
Learn to automate your build, testing, and deployment processes to streamline development.
This practice ensures that code changes are integrated frequently and errors are caught early, leading to faster delivery.
Familiarize yourself with tools like Jenkins, CircleCI, and Travis CI to implement CI/CD in your projects.
Product Recommendations:
• Continuous Delivery by Jez Humble and David Farley
• The DevOps Handbook by Gene Kim
• Accelerate by Nicole Forsgren
10. Refactoring Techniques

Refactoring is the process of restructuring existing code without changing its external behavior.
Regularly refactoring your code can lead to improved performance and maintainability.
Learn techniques for simplifying complex code, eliminating redundancy, and enhancing clarity.
Tools like SonarQube can help identify areas that need refactoring.
Product Recommendations:
• Refactoring: Improving the Design of Existing Code by Martin Fowler
• Clean Architecture by Robert C. Martin
• The Pragmatic Programmer by Andrew Hunt and David Thomas
11. Performance Optimization Techniques
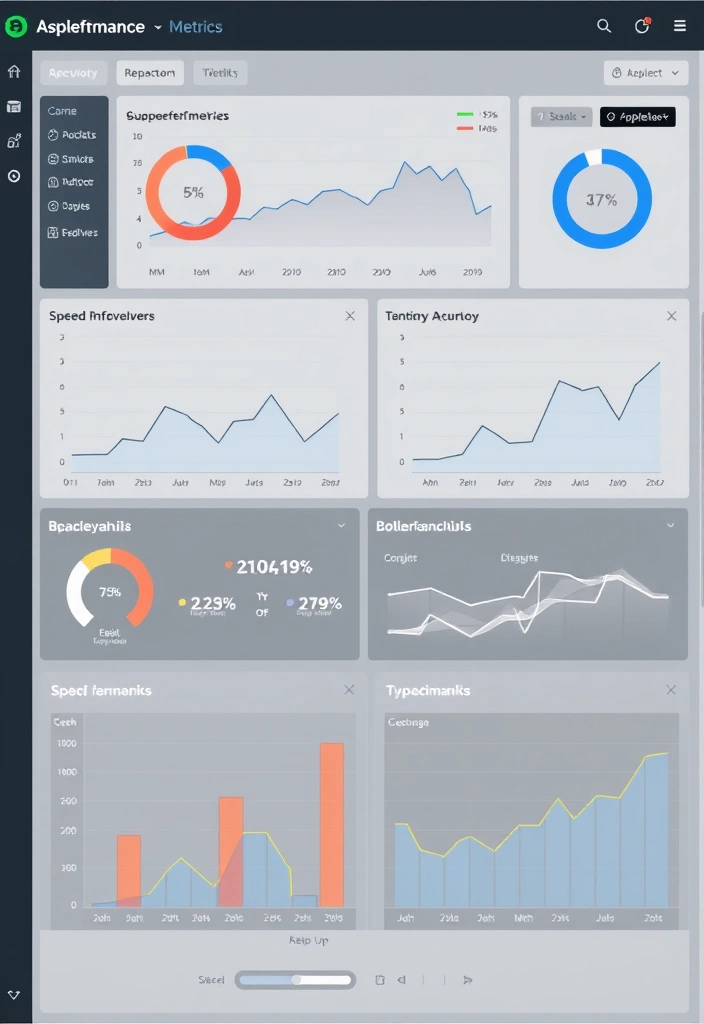
Optimizing your code for performance is essential for handling larger data sets and high traffic.
Explore techniques like code profiling, caching, and database indexing to enhance application speed.
Understanding the performance bottlenecks in your code can lead to significant improvements.
Utilize tools like New Relic, Apache JMeter, or Google Lighthouse to analyze and optimize your applications.
Product Recommendations:
• High Performance Browser Networking by Ilya Grigorik
• Web Performance in Action by Jeremy Wagner
• Performance Analysis in Java by A. U. R. L. D. V. S. D. A. M. K. B. R. R.
12. API Development and Integration
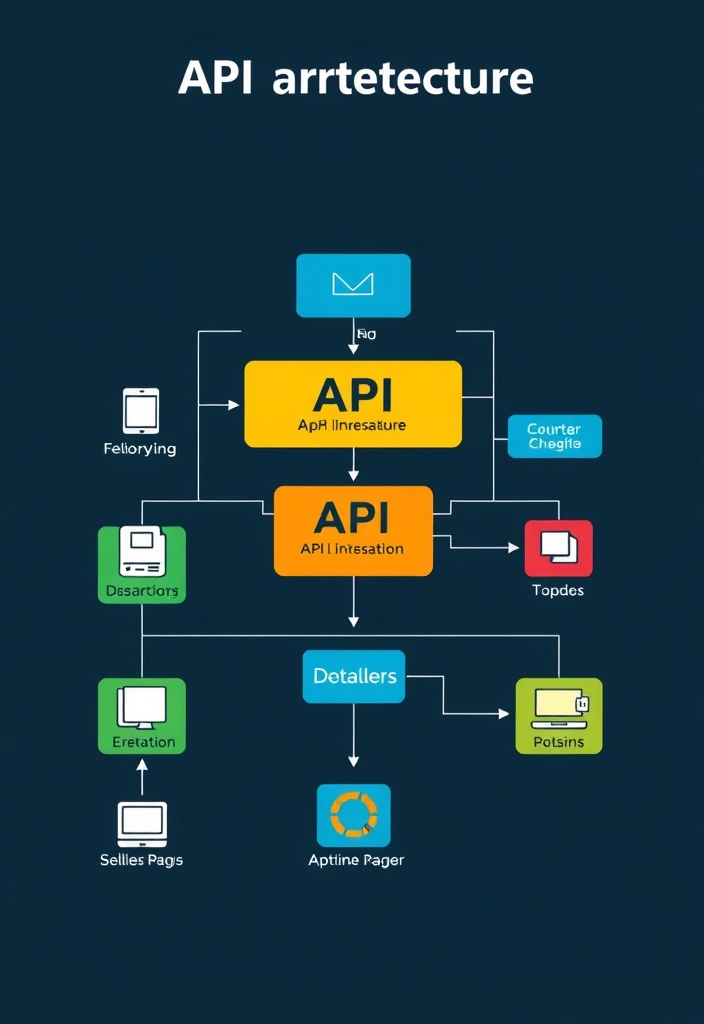
Understanding how to develop and integrate APIs is crucial for modern applications.
Learn RESTful principles and how to use tools like Postman for testing your APIs.
APIs allow different applications to communicate, creating enhanced functionality and user experiences.
Practice designing APIs that are intuitive and well-documented for optimal usability.
Product Recommendations:
• API Design Patterns by JJ Geewax
• RESTful API Design Rulebook by Mark Masse
• Designing Data-Intensive Applications by Martin Kleppmann
13. Security Best Practices
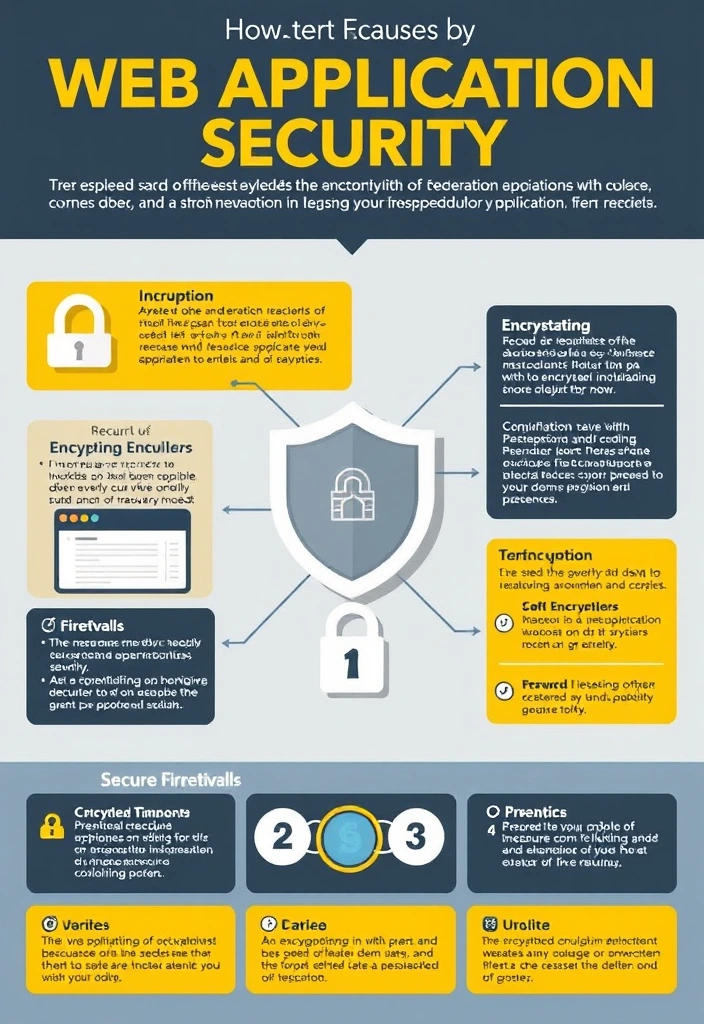
In the age of digital information, security is paramount.
Learn best practices for securing your applications, such as input validation, encryption, and secure authentication methods.
Understanding potential vulnerabilities can help you protect your code and users from attacks.
Regularly update your knowledge on security trends and tools to keep your applications safe.
Product Recommendations:
• The Web Application Hacker’s Handbook by Dafydd Stuttard
• Web Security for Developers by Fortify
14. Data Analysis and Visualization Techniques
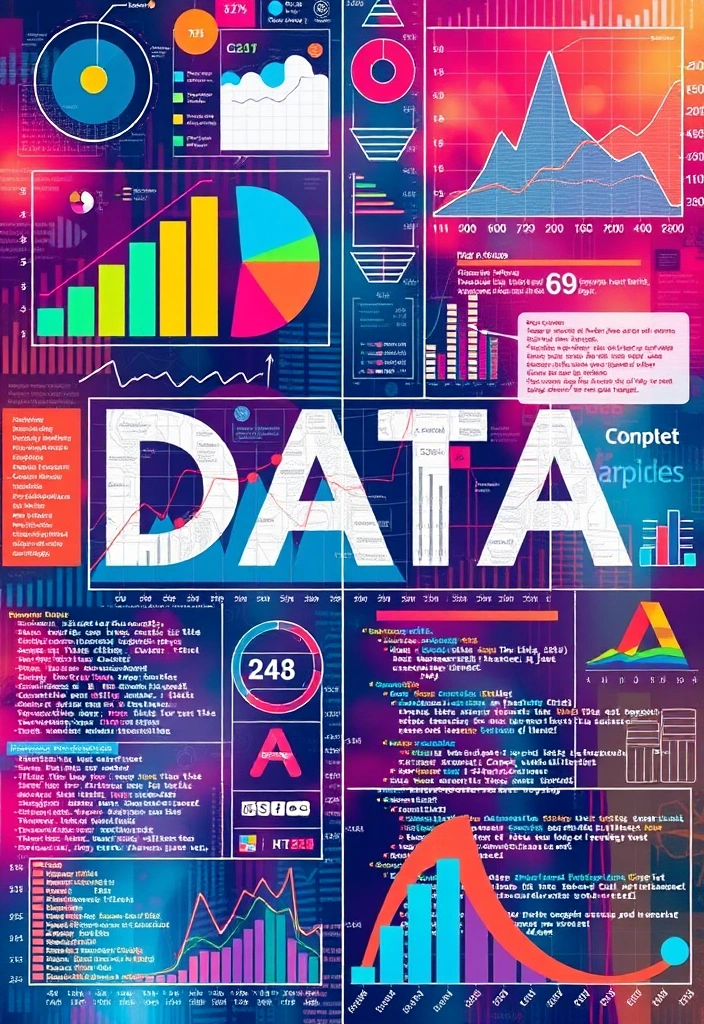
Harness the power of data analysis to make informed decisions in your projects.
Learn to use libraries like Pandas and Matplotlib for analyzing and visualizing data effectively.
Data-driven decision-making can enhance your applications and improve user experience.
Practice creating insightful visualizations that communicate complex data in an understandable way.
Product Recommendations:
• Python for Data Analysis by Wes McKinney
• Data Visualization with Python and Matplotlib by John Murray
• Practical Statistics for Data Scientists by Peter Bruce
15. Leveraging Cloud Services
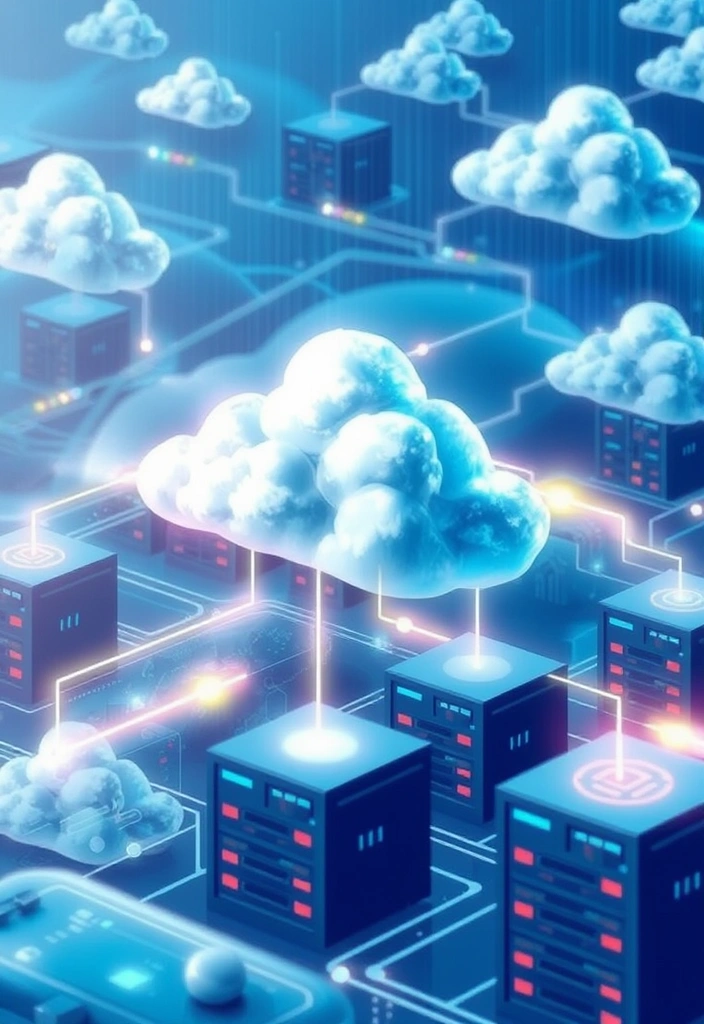
Cloud computing provides powerful resources for scalability and efficiency.
Learn to leverage cloud services like AWS, Google Cloud, or Azure for hosting and deploying applications.
Understanding cloud architecture can significantly enhance your development process and application performance.
Experiment with serverless architecture to simplify deployment and reduce costs.
Product Recommendations:
• Architecting the Cloud by Michael J. Kavis
• AWS Certified Solutions Architect Study Guide by Ben Piper
• Google Cloud Platform in Action by JJ Geewax
16. Mobile Development Techniques
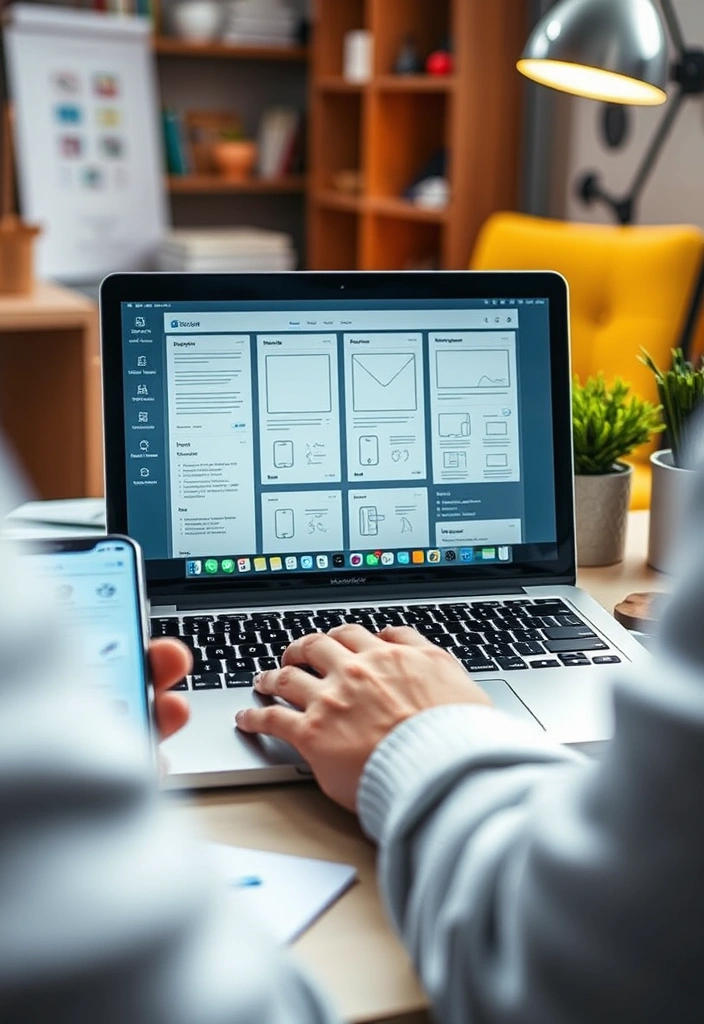
With the rise of mobile applications, understanding mobile development is essential.
Explore frameworks like React Native and Flutter for cross-platform development.
Learn about mobile-specific design principles to create intuitive user experiences.
Regularly update your skills as mobile technology continues to evolve.
Product Recommendations:
• Learning React Native by Bonnie Eisenman
• Flutter for Beginners by Alessandro Biessek
• Mobile App Development with React Native by A. G. P. T. S. D. E. S. S.
17. Game Development Fundamentals

Game development combines creativity with technical skills, making it a unique coding niche.
Explore frameworks like Unity or Unreal Engine to start your journey in game development.
Learn about game mechanics, physics, and graphics design to create engaging experiences.
Practice building small games to hone your skills and understanding.
Product Recommendations:
• Game Programming Patterns by Robert Nystrom
• Unity in Action by Joseph Hocking
• Unreal Engine 4 for Beginners by David Nixon
18. Contributing to Open Source Projects
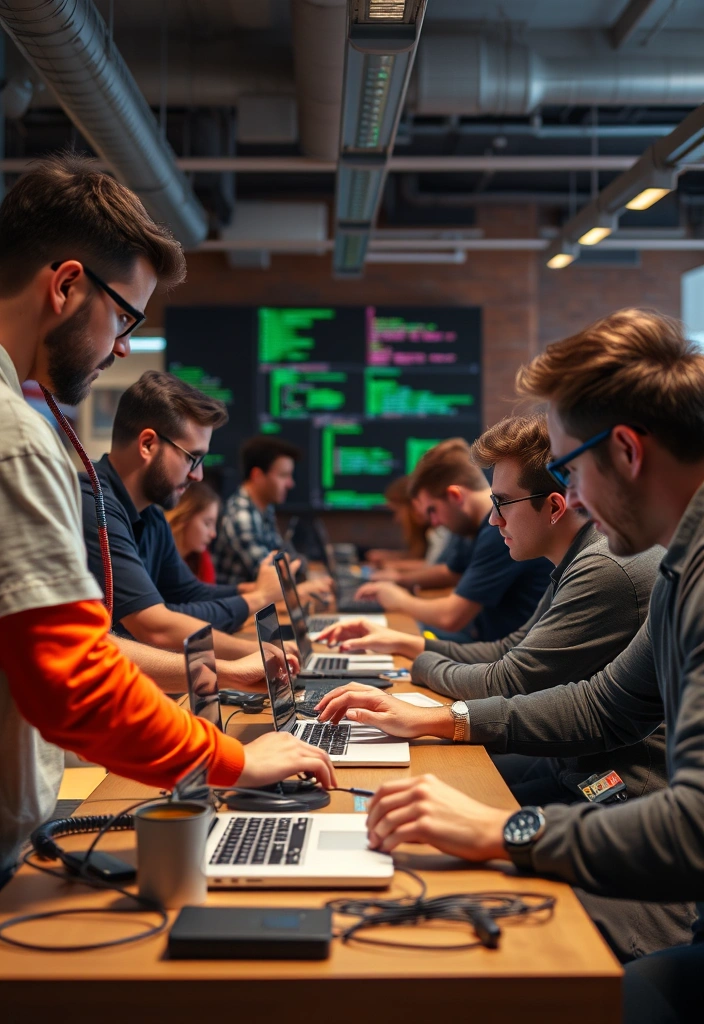
Contributing to open source projects is a fantastic way to learn and grow as a coder.
Engage with communities on platforms like GitHub and start contributing to projects that interest you.
Open source contributions help you build your portfolio and network with other developers.
It’s also an opportunity to learn from experienced coders and improve your skills through collaboration.
Product Recommendations:
• The Art of Community by Jono Bacon
• Producing Open Source Software by Karl Fogel
• Open Source for the Enterprise by Dan Woods
19. Staying Current with Technology Trends
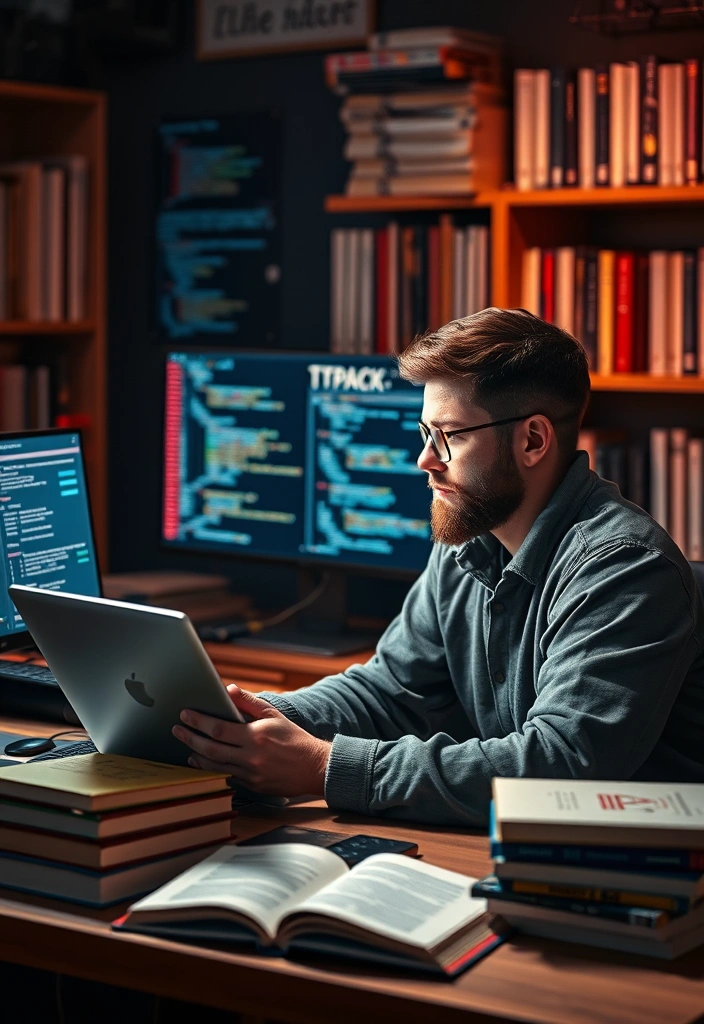
The tech world is ever-evolving, and staying updated is key to remaining relevant.
Follow industry leaders, read tech blogs, and attend webinars to keep your knowledge fresh.
Engaging with current trends can inspire new ideas and improve your coding practices.
Make lifelong learning a part of your coding journey.
Product Recommendations:
• The Innovator’s Dilemma by Clayton M. Christensen
Conclusion

Embracing these advanced coding techniques can transform your approach to programming and enhance your career.
Whether you’re mastering algorithms or diving into mobile development, the journey is filled with opportunities for growth and innovation.
Engage with your community, keep learning, and remember: every great coder started as a beginner.
Note: We aim to provide accurate product links, but some may occasionally expire or become unavailable. If this happens, please search directly on Amazon for the product or a suitable alternative.